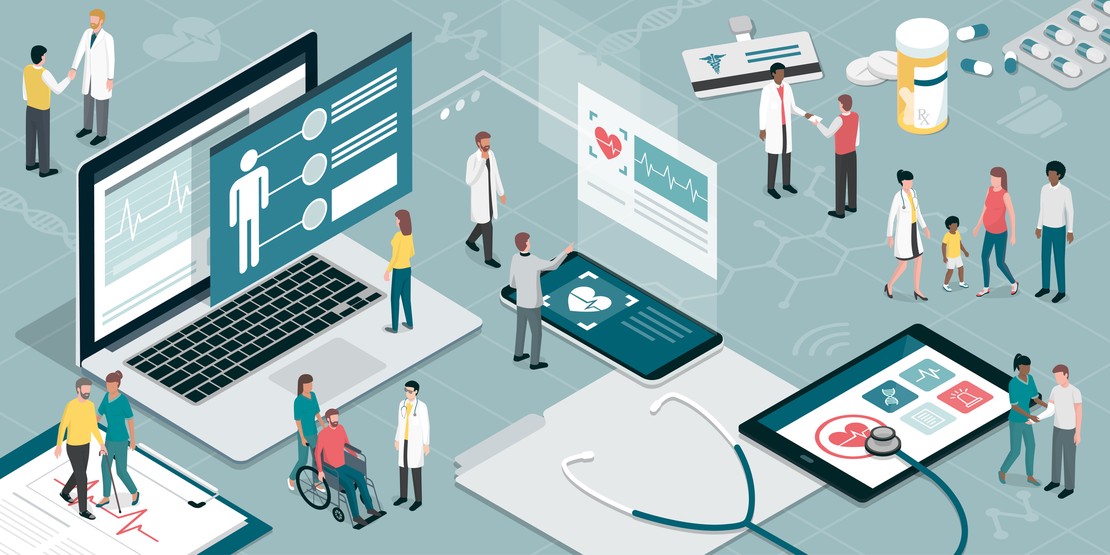
Why the Adapter Pattern is King in Health Data
- Caroline Morton Steph Jones
- Design patterns , Health tech , Health data
- March 9, 2025
Healthcare data is messy. If you’ve ever worked in a clinical setting, you know the frustration logging into multiple systems just to piece together a patient’s history. Pharmacy records don’t sync with GP notes, hospital systems don’t talk to each other, and critical information gets lost in the gaps. In the worst cases, paper documentation is still part of the process.
Recently, a close friend went to a midwife appointment, only to be offered a whooping cough and flu vaccine she had already received two weeks earlier at her GP. The midwife’s system didn’t communicate with the GP’s records. If she hadn’t remembered getting the vaccines, she might have been given an unnecessary second dose. This kind of duplication doesn’t just waste resources-it can lead to medical errors. And this is 2025.
The lack of interoperability in healthcare isn’t just an inconvenience; it has real costs-wasted time (patients and staff), financial inefficiencies, and potential risks to patient safety. Everyone in health tech recognises this challenge, and most software engineers and vendors want to fix it. But turning interoperability from an aspiration into a reality is difficult, especially when working with legacy systems and proprietary formats.
One of the most powerful tools for addressing these challenges is the Adapter Pattern. In this blog, we’ll explore how this design pattern can help bridge the gap between disparate healthcare systems, making integration smoother and more maintainable.
What is a Design Pattern?
Good software design isn’t just about making things work-it’s about making them work well over time. In the 1970s, architect Christopher Alexander introduced the concept of design patterns in building design, describing reusable solutions to common architectural problems. Decades later, software engineers adapted this idea to programming, culminating in the influential book Design Patterns: Elements of Reusable Object-Oriented Software by the Gang of Four in 1994 - a book we highly recommend!
Design patterns act like blueprints for solving recurring software challenges, making code more maintainable, scalable, and adaptable. Without them, every project would feel like reinventing the wheel, leading to inconsistency and technical debt. Just as carpenters rely on tried-and-true joinery techniques to build strong furniture, developers use design patterns to create robust and flexible software.
One reason design patterns are so effective is that they make code more readable and easier to work with. This is where the concept of code beacons, introduced in The Programmer’s Brain , becomes important. Code beacons are structural cues that help developers quickly understand what a piece of code does-whether through meaningful function names, consistent patterns, or well-known abstractions.
For example, when a developer sees an Adapter class, they immediately recognise its role in bridging incompatible interfaces without needing to decipher every line of implementation. This cognitive shortcut reduces onboarding time for new developers and helps them become productive faster when working with a complex codebase.
In healthcare software, where systems often integrate disparate and legacy technologies, leveraging well-known design patterns not only improves the developer experience but also ensures that the software is intuitive, maintainable, and scalable over time.
What is the adapter pattern?
Have you ever traveled to a new country and realised your phone charger doesn’t fit the power outlet? Instead of buying a whole new charger, you just use a plug adapter-a small device that translates between the two formats without changing how your charger or the outlet works. Crucially, the adapter itself isn’t a plug and doesn’t supply power; its only role is to act as an interface, making two otherwise incompatible components work together. In software development, the Adapter Pattern serves the same purpose, acting as a bridge between different systems so they can communicate seamlessly.

Adapters are particularly useful when integrating third-party libraries, legacy code, or systems that were never designed to work together. Instead of rewriting large sections of code, an adapter handles the translation between different interfaces. This aligns with the Single Responsibility Principle-the adapter doesn’t execute business logic or store data; it simply converts data or function calls between incompatible components.
An added benefit is that the adapter allows systems to integrate without modifying their original code. This reduces the risk of unintended side effects-when you change existing code, you might inadvertently break functionality elsewhere that relies on it. In many cases, modifying the original system isn’t even an option, especially when dealing with proprietary or third-party software.
The Adapter Pattern is commonly used in API integrations, especially when one system expects data in JSON while another provides XML, or when different hospital databases store patient records in incompatible formats. Instead of modifying each system, an adapter standardises communication, reducing complexity and technical debt.
Let’s work through an example to really underline the usefulness of this approach.
Python Example: Adapter Pattern for Connecting Two Healthcare Data Systems
To see the Adapter Pattern in action, let’s consider a real-world scenario in healthcare. Different hospital systems often store patient data in incompatible formats, making integration a challenge.
For example, imagine we have:
- System A, which stores patient records in JSON format.
- System B, which expects patient records in XML format.
Rather than rewriting either system, we can use the Adapter Pattern to create an interface that seamlessly converts JSON data into the XML format required by System B-without modifying either system.
Step 1: Define the Incompatible Interfaces
In the Adapter Pattern, the two systems have different interfaces that don’t naturally work together. Here is a shortened version of what each system looks like.
System A: Provides patient data in JSON
class SystemA:
def get_patient_data(self):
return {
"id" : 123,
"name": "Alice Smith",
"age": 45,
"condition": "Hypertension"
}
The method get_patient_data() returns a dictionary (which is essentially JSON).
System B: Requires patient data in XML
class SystemB:
def process_patient_data(self, xml_data):
print(f"Processing patient data in xml format:\n{xml_data}")
This class acts as the “Target Interface” (the system we need to integrate with). The method process_patient_data()
(xml_data) expects XML-formatted data.
Step 2: Implement the Adapter
The Adapter Class will act as a bridge between System A and System B by converting JSON data into XML format.
import xml.etree.ElementTree as ET
class PatientDataAdapter:
def __init__(self, system_a: SystemA):
self.system_a = system_a
def get_patient_data_as_xml(self):
"""Convert JSON data from SystemA to XML format for System B"""
patient_data = self.system_a.get_patient_data()
root = ET.Element("patient")
for key, value in patient_data.items():
child = ET.SubElement(root, key)
child.text = str(value)
return ET.tostring(root, encoding='unicode')
Breaking this down with Adapter Pattern Terminology:
- “Adapter” Class (PatientDataAdapter) - This class acts as the adapter between the incompatible interfaces. It wraps an instance of SystemA and provides a method get_patient_data_as_xml() to convert JSON to XML.
- “Adaptee” (SystemA) - The existing class that provides patient data in JSON format. The adapter does not modify this class-instead, it wraps it.
- “Target Interface” (SystemB) - The format that the adapter must conform to (System B’s XML requirement). The adapter ensures that data from System A is transformed without altering System B.
Step 3: Using the Adapter
Now, let’s see how this works in practice:
system_a = SystemA()
adapter = PatientDataAdapter(system_a)
xml_data = adapter.get_patient_data_as_xml()
system_b = SystemB()
system_b.process_patient_data(xml_data)
Expected Output:
Processing patient data in xml format:
<patient><id>123</id><name>Alice Smith</name><age>45</age><condition>Hypertension</condition></patient>
Why this is a good approach
It maintains the Single Responsibility Principle (SRP) - The adapter only converts formats-it does not alter the behavior of System A or System B.
It enables flexibility - If another system (e.g., System C) needs data in a different format (CSV, YAML, etc.), we can create a different adapter instead of changing System A or System B.
Bridging languages
The Adapter Pattern is also valuable when integrating systems written in different programming languages. In many healthcare systems, electronic prescriptions are generated and stored in Java-based hospital software. However, an analytics system written in Python needs to process this data for trends, compliance tracking, and reporting. Instead of modifying either system, we introduce an adapter that translates Java prescription data into a format the Python analytics system can work with. Java prescription system
In the hospital system, prescription data is stored as a Java object and serialized to JSON before being sent to the analytics system.
import java.io.Serializable;
public class Prescription implements Serializable {
private String patientName;
private String medication;
private int dosage; // Dosage in mg
private String frequency; // e.g., "twice daily"
public Prescription(String patientName, String medication, int dosage, String frequency) {
this.patientName = patientName;
this.medication = medication;
this.dosage = dosage;
this.frequency = frequency;
}
public String toJSON() {
return String.format(
"{\"patientName\": \"%s\", \"medication\": \"%s\", \"dosage\": %d, \"frequency\": \"%s\"}",
patientName, medication, dosage, frequency
);
}
}
Step 2: Python Adapter for Handling Java Data
The Python analytics system receives the JSON prescription data and needs to process it. Since it follows a different naming convention and structure, an adapter is required to transform the incoming Java-style JSON into a Python-friendly format.
import json
class JavaPrescriptionAdapter:
def __init__(self, java_prescription_json):
self.prescription_data = json.loads(java_prescription_json)
def transform(self):
return {
"patient_full_name": self.prescription_data["patientName"],
"medication_name": self.prescription_data["medication"],
"dosage_mg": self.prescription_data["dosage"],
"dose_frequency": self.prescription_data["frequency"]
}
This adapter ensures that the Python analytics system receives data in a format it understands without modifying the original Java prescription system.
Using the system:
# Simulating Java system output
java_prescription_data = '''
{
"patientName": "Alice Smith",
"medication": "Lisinopril",
"dosage": 10,
"frequency": "once daily"
}
'''
# Use the adapter to transform the data
adapter = JavaPrescriptionAdapter(java_prescription_data)
python_prescription_data = adapter.transform()
print(python_prescription_data)
This would produce an output that looks like this.
{
"patient_full_name": "Alice Smith",
"medication_name": "Lisinopril",
"dosage_mg": 10,
"dose_frequency": "once daily"
}
If in the future we decide to use R instead of Python, or if we need to integrate with an off-the-shelf analytics solution like Tableau, we can create new adapters rather than rewriting core logic. The first adapter handles Java to Python, and future adapters can be written for specific new use cases.
Base Adapters
A scalable approach we have used is to define a base adapter class in Python using abstract classes. This ensures that all adapters follow a common structure while allowing flexibility for different target systems.
Using Python’s abc module, we can create a base adapter that enforces a standard structure for data transformation.
from abc import ABC, abstractmethod
import json
class BasePrescriptionAdapter(ABC):
def __init__(self, java_prescription_json):
self.prescription_data = json.loads(java_prescription_json)
@abstractmethod
def transform(self):
"""Method that must be implemented by all subclasses to format prescription data."""
pass
This base class ensures that any new adapter must implement a transform method. This base class ensures that any new adapter must implement a transform method. Now, let’s define two concrete implementations-one for analytics and another for pharmacy stock tracking
Adapter for Analytics (PythonPrescriptionAnalyticAdapter)
class PythonPrescriptionAnalyticAdapter(BasePrescriptionAdapter):
def transform(self):
return {
"patient_full_name": self.prescription_data["patientName"],
"medication_name": self.prescription_data["medication"],
"dosage_mg": self.prescription_data["dosage"],
"dose_frequency": self.prescription_data["frequency"]
}
Our adapter inherits from the base adapter and implements the transform method, and allows our analytic platform to take this as an input.
Imagine that the hospital pharmacy also wants to track prescriptions so they can make sure their stock is maintained. Instead of rewriting transformation logic, we can extend the base adapter and create a specialised adapter for inventory management.
class PharmacyStockAdapter(BasePrescriptionAdapter):
def transform(self):
return {
"medication": self.prescription_data["medication"],
"dosage_mg": self.prescription_data["dosage"],
"quantity_prescribed": 1,
"needs_restock": self.prescription_data["dosage"] > 100
}
This adapter allows a pharmacy system to track medication stock and decide whether a reorder is needed. We can use it like this:
# Create and use the Pharmacy Stock Adapter
pharmacy_adapter = PharmacyStockAdapter(java_prescription_data) pharmacy_stock_data = pharmacy_adapter.transform()
print(pharmacy_stock_data)
This outputs a different output to the JavaPrescriptionAdapter:
{
"medication": "Lisinopril",
"dosage_mg": 10,
"quantity_prescribed": 1,
"needs_restock": False
}
As you can see, the pharmacy system receives only the medication details relevant to inventory management, with an added flag indicating whether the medication needs restocking. This allows the pharmacy system to maintain appropriate stock levels without needing to understand the full patient prescription context.
Conclusion
The adapter pattern is a practical solution for integrating incompatible systems in healthcare. Instead of modifying core systems, an adapter translates data formats while keeping each system loosely coupled and easy to maintain.
By implementing a base adapter structure, healthcare software can scale without unnecessary complexity, allowing new integrations without rewriting core logic. If a new system, such as an insurance claims processor or a compliance monitoring tool, requires prescription data in a different format, a new adapter can be introduced seamlessly.
This approach reduces technical debt, improves maintainability, and allows healthcare systems to focus on providing better patient care rather than wrestling with incompatible data formats.